Titles Customizing the Title Metadata Shown in Browser Tabs
In this demonstration, I will show how to customize the title metadata of webpages in an existing Rails web app. The title metadata for a webpage commonly appears in the tab of the web browser, as illustrated in Figure 1.
General Steps
In general, the steps for customizing the title metadata of webpages in an existing Rails-based web app are as follows.
-
Step 1 Set a Global Title for the Web App. This step sets a global title for all of the app’s webpages.
-
Step 2 Add Page-Specific Titles. This step enables an individual webpage to optionally specify its own page-specific title.
Adding Custom Webpage Titles to the Billy Books App
To demonstrate the steps for customizing the title of a webpage, we will be customizing the titles of the webpages in the Billy Books bookstore base app. The base app has two webpages: a home page and an about page.
We will customize the title of the home page to be the name of the web app, “Billy Books”, as illustrated in Figure 1.
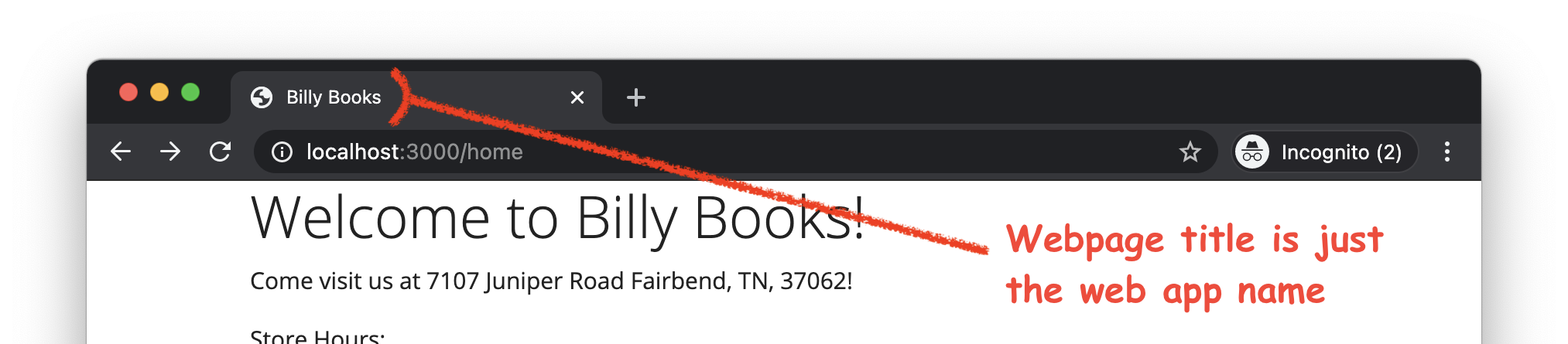
Figure 1. The Billy Books home page with the title “Billy Books” in the browser tab.
We will customize the title of the about page to include both a webpage-specific part, “About Us”, as well as the name of the web app, as illustrated in Figure 2.
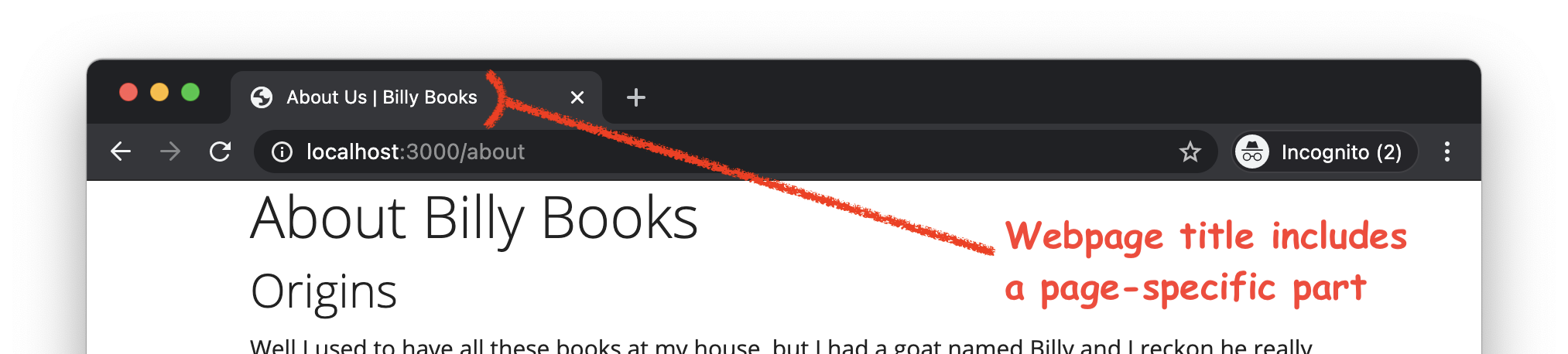
Figure 2. The Billy Books about page with the page-specific title “About Us” in the browser tab.
Step 1 Set a Global Title for the Web App
When we run the base app and look at the title bar, we see that the title of the app is currently “RailsDemosNDeets2021App”. This is the default title created by the rails new
command used to create the web app initially.
This title value was specified in the HTML.ERB view layout, app/views/layouts/application.html.erb
. Specifically, it is in the content of the HTML <title>
element, which looks like this:
<head>
<title>RailsDemosNDeets2021App</title>
…
</head>
To globally change the title of all of the app’s webpages to “Billy Books”, we edit the content of the <title>
element in app/views/layouts/application.html.erb
, like this:
<head>
<title>Billy Books</title>
…
</head>
Test It!
To confirm that we made this change correctly, we run the web app (as per the steps in the running apps demo), and we open the home page (http://localhost:3000/home) in our web browser. The webpage title in the browser tab should say “Billy Books”, as shown in Figure 1.
Note that if we open the about page (http://localhost:3000/about), it will also display only “Billy Books” in the browser tab. Next, we will add a webpage-specific part to the about page’s title.
Step 2 Add Page-Specific Titles
Substep
Declare webpage-specific titles in view templates. To add a webpage-specific title to the Billy Books about page, we first declare the about page’s title at the top of the page’s HTML.ERB view template, app/views/pages/about.html.erb
, like this:
<% content_for(:html_title) { 'About Us' } %>
<h1>About Billy Books</h1>
…
This declaration stores what is called a view partial in Rails. In this case, the partial contains the text “About Us” and is mapped to the symbol :html_title
, which can be used to retrieve the partial later. We can add a declaration like this to the view for each webpage that we want to have a page-specific title.
Substep
Render the webpage-specific title in the layout. To make the page-specific titles render in the appropriate pages, we add a yield
statement to the <title>
element in the view layout, app/views/layouts/application.html.erb
, like this:
<head>
<title>
<%= yield(:html_title) %> | Billy Books
</title>
…
</head>
Now, when a view template (like app/views/pages/about.html.erb
) renders, its :html_title
partial will be retrieved by the above call to yield
and included in the webpage title.
This change leaves one remaining bug that we must fix: view templates that don’t declare an :html_title
partial will receive the title “| Billy Books”, with an extraneous bar (“|”) separator.
Substep
Handle cases in which no webpage-specific title was declared. To fix this bug, we add conditional logic to app/views/layouts/application.html.erb
so that the page-specific title and separator are included only if an :html_title
partial was declared, like this:
<head>
<title>
<% if content_for?(:html_title) %>
<%= yield(:html_title) %> |
<% end %>
Billy Books
</title>
…
</head>
Test It!
To confirm that we made this change correctly, we run the web app (as per the steps in the running apps demo), and we open the about page (http://localhost:3000/about) in our web browser. The webpage title in the browser tab should say “About Us | Billy Books”, including the webpage-specific title, as shown in Figure 2. Furthermore, when we open the home page (http://localhost:3000/home) in our web browser, we should see that the title remains on “Billy Books”, as shown in Figure 1.
Conclusion
Following the above steps, we have now customized the title metadata for the pages of the Billy Books bookstore app, so that the default page title is the name of the bookstore and page-specific titles can be optionally specified in the page’s view template.