Running Apps Initializing and Executing the Demo Apps
In this demo, I will show how to download, initialize, and run the example Rails web apps used in these demos.
General Steps
In general, the steps for downloading, initializing, and running the demo apps are as follows.
-
Step 1 Clone the GitHub Repo (if Needed). All the demo app source code is stored in a Git repository (repo for short) that is hosted on GitHub. This step downloads a copy of the Git repo to our computer.
-
Step 2 Switch Branches (if Needed). The Git repo is organized into different named branches, and each branch corresponds to a different demo app (or a different version of a demo app). This step switches the local working directory so that it contains the code for the appropriate demo app.
-
Step 3 Install the Ruby Gem Dependencies. Any Rails web app project will have numerous Ruby gems (i.e., code libraries) that it depends on. This step downloads all the required gems, so the project can use them.
-
Step 4 Install the JavaScript Dependencies. Modern web apps commonly make heavy use of JavaScript and depend on many JavaScript packages (i.e., code libraries). This step downloads all the required JavaScript packages, so the project can use them.
-
Step 5 Initialize the Database. Most of the demo web apps will make use of a database backend for saving and managing data. This step initializes the database to prepare it for use by the app, and it populates the database with seed data if the app has any.
-
Step 6 Run the Development Web Server. This step starts the Rails development webserver, effectively running the app and enabling it to be accessed via a web browser.
Running the Team Roster App
To demonstrate the steps for initializing and executing an app, we will be running a team-roster app for managing player data for a basketball team.
Step 1 Clone the GitHub Repo
To download a copy of demo apps repo, we run the git clone
command, like this:
git clone git@github.com:human-se/rails-demos-n-deets-2021-app.git
As a result, a folder named rails-demos-n-deets-2021-app
will be created in the current directory, and the folder will serve as a working directory containing a version of the code for one of the demos (the demo on the repo’s main
branch initially) and a (hidden) local repo (i.e., database) of all the demos and their version histories, which can be accessed via the git
command.
If we want to folder created in the current directory to have a different name than rails-demos-n-deets-2021-app
, we can an alternative version of the command that adds the name we want onto the end. For example, if we wanted the folder to be named demo_app
, we could run this command:
git clone git@github.com:human-se/rails-demos-n-deets-2021-app.git demo_app
The result of this command will be exactly the same as before, except the folder created will be named demo_app
.
Test It!
To confirm that we cloned the repo correctly, we cd
into the folder, and we display its contents, like this:
ls -a1
The output should look like this:
.
..
.browserslistrc
.git
.gitattributes
.gitignore
.ruby-version
Gemfile
Gemfile.lock
README.md
Rakefile
app
babel.config.js
bin
config
config.ru
db
lib
log
package.json
postcss.config.js
public
storage
test
tmp
vendor
yarn.lock
Additionally, we can confirm the status of the local repo with respect to Git, like this:
git status
The output should look like this:
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
Note that the output indicates that we are currently on the main
branch.
Step 2 Switch Branches
After you have cd’d into the project folder with the command cd rails-demos-n-deets-2021-app
(or cd demo_app
if you used the second command above), you can switch branches. The team-roster app code is on the branch demo-scaffolds
, but we are currently on the main
branch. To switch branches, we run the git switch
command, like this:
git switch demo-scaffolds
The output of the command should look like this:
Branch 'demo-scaffolds' set up to track remote branch 'demo-scaffolds' from 'origin'.
Switched to a new branch 'demo-scaffolds'
Coping with Errors Caused by an Unclean Working Directory
If the git switch
command instead produces an error message, like this:
error: Your local changes to the following files would be overwritten by checkout: …
or if it outputs one or more lines beginning with M
, like this:
M path/to/some.file
then we somehow have uncommitted/untracked changes in our working directory. The reasons why such changes would present vary, but in any case, we will need to do something about them. If we simply want to erase them, we can perform the following steps.
To delete all untracked files, we run the git clean
command, like this:
git clean -df
To undo all changes made to tracked files (includes both staged and unstaged changes), we run the git restore
command, like this:
git restore --worktree --staged :/
Finally, if the previous git switch
command failed with the error:…
message, then we will need to run it again on our now-clean working directory.
Test It!
To confirm that we switched branches correctly, we check the status of the Git repo, like this:
git status
The output of the command should look like this:
On branch demo-scaffolds
Your branch is up to date with 'origin/demo-scaffolds'.
nothing to commit, working tree clean
Step 3 Install the Ruby Gem Dependencies
To install all the Gem dependencies for the app, we run the bundle
command, like this:
bundle install
The command may take some time to complete, and it should produce a long list of output that tells which of the required gems it installed and which ones were already installed.
Step 4 Install the JavaScript Dependencies
To install all the JavaScript package dependencies for the app, we run the yarn
command, like this:
yarn install
The command may take some time to complete, and the output of the command should look like this:
yarn install v1.22.10
[1/4] 🔍 Resolving packages...
[2/4] 🚚 Fetching packages...
[3/4] 🔗 Linking dependencies...
warning " > webpack-dev-server@3.11.2" has unmet peer dependency "webpack@^4.0.0 || ^5.0.0".
warning "webpack-dev-server > webpack-dev-middleware@3.7.3" has unmet peer dependency "webpack@^4.0.0 || ^5.0.0".
[4/4] 🔨 Building fresh packages...
✨ Done in 159.30s.
Additionally, a new folder, node_modules
, which contains all of the JS packages, should have been created in the top-level folder of the project’s working directory.
Step 5 Initialize the Database
To (re-)initialize the database and populate it with seed data, we run the rails
command, like this:
rails db:migrate:reset db:seed
The output should look like this:
Dropped database 'rails_demos_n_deets_2021_app_development'
Dropped database 'rails_demos_n_deets_2021_app_test'
Created database 'rails_demos_n_deets_2021_app_development'
Created database 'rails_demos_n_deets_2021_app_test'
== 20210118230130 CreateBasketBallPlayers: migrating ==========================
-- create_table(:basket_ball_players)
-> 0.0090s
== 20210118230130 CreateBasketBallPlayers: migrated (0.0091s) =================
Model files unchanged.
Test It!
To confirm that the database was initialized and seeded correctly, we use the Rails console to test it out.
To start the console, we run the rails
command, like this:
rails console
To confirm that the seed data was saved to the database, we call the Car
model class method all
, like this:
pp BasketBallPlayer.find(4)
The pp
method “pretty prints” the object passed as its argument, making it easier to inspect the object. In this case, the argument passed to pp
is the BasketBallPlayer
object with an ID of 4 retrieved from the database.
The console output should look like this:
BasketBallPlayer Load (0.3ms) SELECT "basket_ball_players".* FROM "basket_ball_players" WHERE "basket_ball_players"."id" = $1 LIMIT $2 [["id", 4], ["LIMIT", 1]]
#<BasketBallPlayer:0x00007fc0529c1638
id: 4,
first_name: "Landers",
last_name: "Nolley II",
position: "Guard",
height_inches: 79,
weight_lbs: 220,
created_at: Tue, 19 Jan 2021 03:54:13.597001000 UTC +00:00,
updated_at: Tue, 19 Jan 2021 03:54:13.597001000 UTC +00:00>
=> #<BasketBallPlayer id: 4, first_name: "Landers", last_name: "Nolley II", position: "Guard", height_inches: 79, weight_lbs: 220, created_at: "2021-01-19 03:54:13.597001000 +0000", updated_at: "2021-01-19 03:54:13.597001000 +0000">
Note that, as we would expect, the fourth BasketBallPlayer
object created in the seeds script was retrieved by the call to find
.
To wrap up, we quit the Rails console by entering this command:
exit
Step 6 Run the Development Web Server
To start the development webserver that runs the demo app, we run the rails
command, like this:
rails server
The output should look like this:
=> Booting Puma
=> Rails 6.1.1 application starting in development
=> Run `bin/rails server --help` for more startup options
Puma starting in single mode...
* Puma version: 5.1.1 (ruby 2.7.2-p137) ("At Your Service")
* Min threads: 5
* Max threads: 5
* Environment: development
* PID: 67260
* Listening on http://127.0.0.1:3000
* Listening on http://[::1]:3000
Use Ctrl-C to stop
Note that, while the server is running, the command line is tied up such that no other commands can be run.
To halt the web server and free up the command line, type CTRL-C. (Note that even Mac users must use the CTRL/control key and not the Mac’s command key.)
Test It!
To verify that the web app is running correctly, we open the URL http://localhost:3000/basket_ball_players in our web browser. The webpage displayed should look exactly like Figure 1.
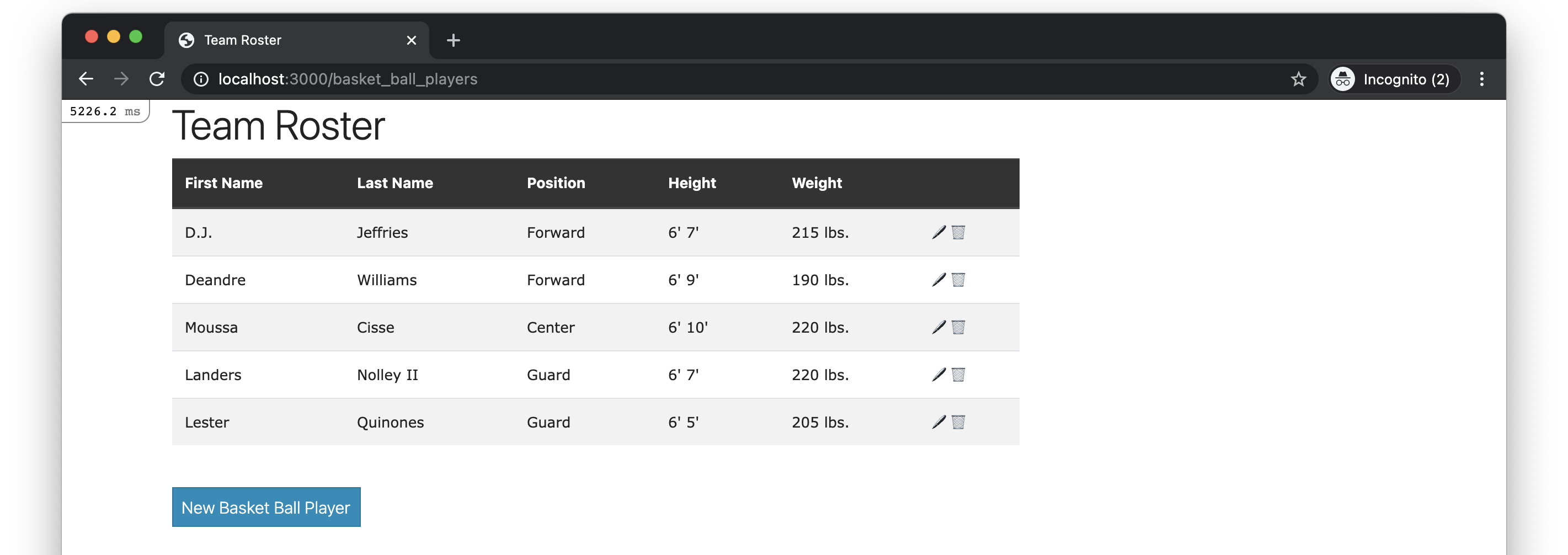
Figure 1. The team-roster app.
Additionally, we try creating, editing, and deleting player entries to make sure that the database backend is functioning correctly.
Conclusion
Following the above steps, we have now run the team-roster demo app. However, the general steps performed in this demo can be used to run any of the demo apps.