New Projects Generating and Configuring a New Rails Web App
In this demonstration, I will show you how to create and setup a new Rails web app project.
General Steps
In general, the steps for creating and setting up a Rails-based web app are as follows.
-
Step 1 Create a Named Gemset. This step uses RVM to create a gemset (think of it as a special system folder) where all the Ruby gems used by the project will be stored. Because different projects use different gems and different versions thereof, it is helpful to keep the gems for different projects separated in their own project-specific gemsets.
-
Step 2 Generate a New Rails Project. This step uses Rails to generate the basic code skeleton for a new web app. This code provides a foundation upon which the features of an app can be built.
-
Step 3 Set the Project’s Ruby Version and Gemset. This step adds settings to the app that tell RVM what version of Ruby is required for the app and the name of the gemset. Having this configuration is useful for a couple reasons: collaborators won’t accidentally try to use an incompatible version of Ruby on the project, and RVM will know to automatically create the appropriate gemset for the project on the collaborator’s system.
-
Step 4 Add Ruby Gems (if Needed). This step adds additional Ruby gems that are needed for the project, but did not come with it by default.
-
Step 5 Add JavaScript Packages (if Needed). This step adds JavaScript (JS) packages that provide additional features and support for building the interface of the app.
Creating the Base App for All Demos
To demonstrate the steps for creating and setting up a web app, we will be making the base app upon which all the example apps used in the demos are built. We will call the project, rails-demos-n-deets-2021-app
.
Step 1 Create a Named Gemset
To create a gemset named rails-6-app
in which all the project’s Ruby gem dependencies will be housed, we run the rvm
command, like this:
rvm use 2.7.2@rails-6-app --create
Test It!
To confirm that the gemset was created correctly, we run the rvm
command, like this:
rvm gemset list
The output should include this line:
=> rails-6-app
The =>
indicates the gemset currently being used, which should be the gemset we just created, rails-6-app
.
Step 2 Generate a New Rails Project
To generate an initial skeleton for the rails-demos-n-deets-2021-app
app, we run the rails new
command, like this:
rails new rails-demos-n-deets-2021-app --database=postgresql
Note that, in the above command, the rails-demos-n-deets-2021-app
argument specifies the name of the app to be generated, and the --database=postgresql
option specifies that the app will use a PostgeSQL DBMS for its backend (as opposed to the default, SQLite).
The command produces a considerable amount of output, and it takes some time to run. First, it outputs a number of create
line, indicating the project files being generated. These lines will be followed by a number of Fetching
/Installing
/Using
lines, indicating the Ruby gems that are being automatically added to the project. Finally, the JavaScript packages will be installed, resulting in another long list of package names and versions, and concluding with this message:
Webpacker successfully installed 🎉 🍰
As a result of the command, a new folder, rails-demos-n-deets-2021-app
, should have been created in the current directory.
Important! We do not cd
into the generated folder until completing the next step, which sets the Ruby version and gemset.
Test It!
To at least partially verify that the above command ran correctly, we manually inspect the command’s output, looking for any fatal-looking errors (as opposed to benign warnings), and we do an ls
to see that the rails-demos-n-deets-2021-app
folder was added to the current directory, as expected.
Step 3 Set the Project’s Ruby Version and Gemset
To add a setting to the app that will ensure that the correct version of Ruby and the rails-6-app will always be used with it, we open the project in VS Code by running this command:
code rails-demos-n-deets-2021-app
Then, in VS Code, we open the file, .ruby-version
, and we edit it, like this:
2.7.2@rails-6-app
This file will ensure that whenever a user does a cd
into the project folder, RVM will use the specified version of Ruby (2.7.2
) and the specified gemset (rails-6-app
), creating the gemset if necessary.
Test It!
To confirm that this setting is working correctly, we first switch gemsets to the default gemsets by running this command:
rvm use 2.7.2
Then, we cd
into the project folder. The gemset should automatically switch to rails-6-app
. To confirm that it changed correctly, we run the rvm
command, like this:
rvm gemset list
The output should include this line, indicating that we are currently using the correct gemset:
=> rails-6-app
Step 4 Add Ruby Gems
In general, the Ruby gems used in an app will vary, depending on the app’s specific needs, and the steps for adding gems will vary from gem to gem. Below, we add the gems that will be used in all the example apps from the demos.
Substep
Add the pgreset gem. The pgreset gem resolves some errors that we may get when resetting our PostgreSQL database while working on the project. To install the pgreset gem, we open the project’s Gemfile
in VS Code, and we add the following lines to the end of the file:
# Disconnects all connections to PostgreSQL db when running rails db:reset
gem 'pgreset'
Then, we run the bundle
command to complete the installation, like this:
bundle install
Substep
Add the Annotate gem. The Annotate gem automatically adds comments to each model class file, listing the model’s attributes and their types. To install the Annotate gem, we add the following to the end of the project’s Gemfile
:
group :development do
gem 'annotate'
end
Then, we run the bundle
command to complete the gem installation, like this:
bundle install
Finally, Annotate requires an additional step to set up properly: we run the following command in the terminal in the project folder:
rails generate annotate:install
The output should look like this:
Running via Spring preloader in process 16531
create lib/tasks/auto_annotate_models.rake
Substep
Add the bootstrap_form gem. The bootstrap_form gem simplifies the code required to implement forms in a Rails project. To install this gem, we first add the following to the end of the project’s Gemfile
:
# Replaces Rails form helpers with bootstrap-specific form helpers
gem "bootstrap_form", "~> 4.0"
Then, we run the bundle
command to complete the gem installation, like this:
bundle install
Finally, to integrate the gem into the app, we add some stylesheet code.
First, in the project folder, app/assets/stylesheets
, we rename the file, application.css
, to be application.scss
(note the extra s
in the scss
suffix). We can rename the file using VS Code or using the mv
command (run from the top-level project folder), like this:
mv app/assets/stylesheets/application.css app/assets/stylesheets/application.scss
Then, we edit the application.scss
, insert an @import
line at the bottom of the file, like this:
/*
* This is a manifest file that'll be compiled into application.css, which will include all the files
* listed below.
*
* Any CSS and SCSS file within this directory, lib/assets/stylesheets, or any plugin's
* vendor/assets/stylesheets directory can be referenced here using a relative path.
*
* You're free to add application-wide styles to this file and they'll appear at the bottom of the
* compiled file so the styles you add here take precedence over styles defined in any other CSS/SCSS
* files in this directory. Styles in this file should be added after the last require_* statement.
* It is generally better to create a new file per style scope.
*
*= require_tree .
*= require_self
*/
@import "rails_bootstrap_forms";
Test It!
To confirm that we performed the above steps correctly, we run the app by performing the following steps, which were described in more detail in the running apps demo).
Since we already installed the Ruby gems and JavaScript packages above, we can skip those steps here.
To initialize the database, we run the rails
command, like this:
rails db:migrate:reset db:seed
The output should look like this:
Dropped database 'rails_demos_n_deets_2021_app_development'
Dropped database 'rails_demos_n_deets_2021_app_test'
Created database 'rails_demos_n_deets_2021_app_development'
Created database 'rails_demos_n_deets_2021_app_test'
Model files unchanged.
To start the development server, we run the rails
command, like this:
rails server
To try out the app, we open the URL http://localhost:3000/ in a web browser. The page displayed should look like Figure 1.
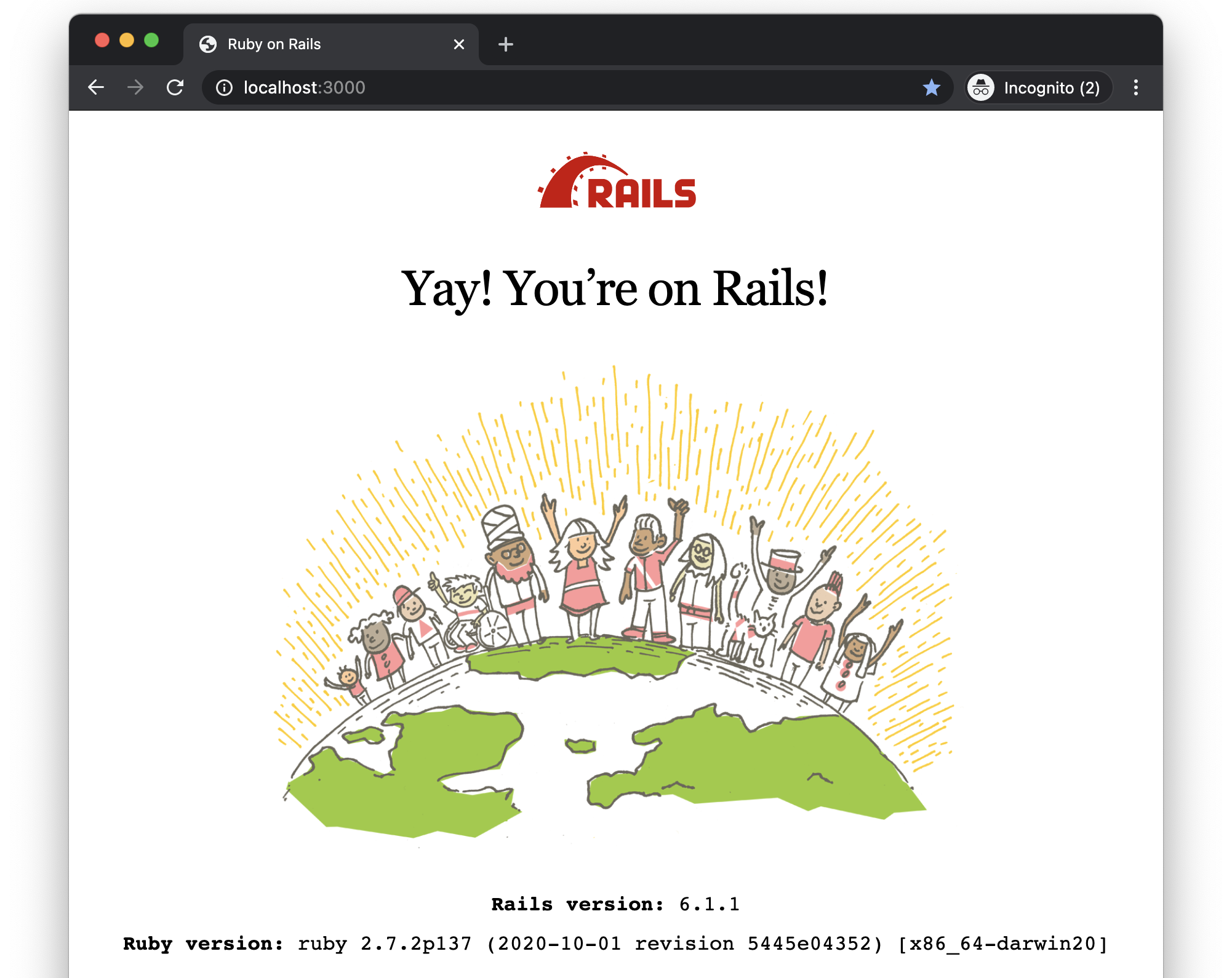
Figure 1. The Rails default landing page.
Step 5 Add JavaScript Packages
In general, the JS packages used in an app will vary, depending on the app’s specific needs, and the steps for adding JS packages will vary from package to package. Below, we add the JS packages that will be used in all the example apps from the demos.
Substep
Add the Bootstrap package. Bootstrap is a popular toolkit for creating stylish, modern-looking webpages. To install Bootstrap, we first run the yarn add
command, like this:
yarn add bootstrap jquery popper.js
This command will install Bootstrap and its two dependencies, jQuery and Popper.
To integrate Boostrap into the app, we must import the Bootstrap JS and CSS code.
To integrate the JS code, we open the file, app/javascript/packs/application.js
in VS Code, and we add an import
line to the bottom of the file, like this:
// This file is automatically compiled by Webpack, along with any other files
// present in this directory. You're encouraged to place your actual application logic in
// a relevant structure within app/javascript and only use these pack files to reference
// that code so it'll be compiled.
import Rails from "@rails/ujs"
import Turbolinks from "turbolinks"
import * as ActiveStorage from "@rails/activestorage"
import "channels"
Rails.start()
Turbolinks.start()
ActiveStorage.start()
import 'bootstrap'
To integrate the CSS code, we open the file, app/assets/stylesheets/application.scss
in VS Code, and we add an @import
line to the bottom of the file, like this:
/*
* This is a manifest file that'll be compiled into application.css, which will include all the files
* listed below.
*
* …
*
*= require_tree .
*= require_self
*/
@import "rails_bootstrap_forms";
@import "bootstrap/scss/bootstrap";
In addition to doing the above imports, a couple more changes are needed to fully integrate Bootstrap into the project.
To provide shortcuts to every JS file for jQuery and Popper, we add code to the bottom of the file, config/webpack/environment.js
, like this:
const { environment } = require('@rails/webpacker')
module.exports = environment
const webpack = require('webpack')
environment.plugins.prepend('Provide',
new webpack.ProvidePlugin({
$: 'jquery',
Popper: ['popper.js', 'default']
})
)
To enable Bootstrap’s tooltip and popover components to work properly, we add code to the bottom of the file, app/javascript/packs/application.js
, like this:
// This file is automatically compiled by Webpack, along with any other files
// present in this directory. You're encouraged to place your actual application logic in
// a relevant structure within app/javascript and only use these pack files to reference
// that code so it'll be compiled.
import Rails from "@rails/ujs"
import Turbolinks from "turbolinks"
import * as ActiveStorage from "@rails/activestorage"
import "channels"
Rails.start()
Turbolinks.start()
ActiveStorage.start()
import 'bootstrap'
document.addEventListener("turbolinks:load", () => {
$('[data-toggle="tooltip"]').tooltip();
$('[data-toggle="popover"]').popover();
})
Finally, to apply the basic Bootstrap page layout all pages of the app, we insert a <main>
element into the view layout, app/views/layouts/application.html.erb
, like this:
<!DOCTYPE html>
<html>
<head>
<title>RailsDemosNDeets2021App</title>
<meta name="viewport" content="width=device-width,initial-scale=1">
<%= csrf_meta_tags %>
<%= csp_meta_tag %>
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
<%= javascript_pack_tag 'application', 'data-turbolinks-track': 'reload' %>
</head>
<body>
<main role="main" class="container">
<%= yield %>
</main>
</body>
</html>
Substep
Add the Bootswatch package. Bootswatch is a package that uses the Bootstrap CSS classes and organizes them into app-wide themes that can easily be switched out and changed. To install Bootswatch, we first run the yarn add
command, like this:
yarn add bootswatch
To integrate Bootswatch into the project, we add two new @import
statements to the file, app/assets/stylesheets/application.scss
, like this:
/*
* This is a manifest file that'll be compiled into application.css, which will include all the files
* listed below.
*
* …
*
*= require_tree .
*= require_self
*/
@import "rails_bootstrap_forms";
@import "bootswatch/dist/yeti/variables";
@import "bootstrap/scss/bootstrap";
@import "bootswatch/dist/yeti/bootswatch";
Caution! It important that the variables
line goes before the bootstrap
line in order for this code to work correctly.
The word yeti
that appears in each of the two new @import
statements refers to the Bootswatch Yeti theme. Other themes can be found on the Bootswatch website, and we can apply one of these other themes to the project by changing the word yeti
in these @import
lines to the name of a different theme.
Substep
Add the Autosize package. The Autosize package is used to make textarea form fields that automatically adjust their height based on the amount of text that a user enters into them. To install the Autosize package, we run the yarn add
command, like this
yarn add autosize
To integrate Autosize into the project, we insert an import
statement and a call to the autosize
function into the file, app/javascript/packs/application.js
, like this:
// This file is automatically compiled by Webpack, along with any other files
// present in this directory. You're encouraged to place your actual application logic in
// a relevant structure within app/javascript and only use these pack files to reference
// that code so it'll be compiled.
import Rails from "@rails/ujs"
import Turbolinks from "turbolinks"
import * as ActiveStorage from "@rails/activestorage"
import "channels"
Rails.start()
Turbolinks.start()
ActiveStorage.start()
import 'bootstrap'
import 'autosize'
document.addEventListener("turbolinks:load", () => {
$('[data-toggle="tooltip"]').tooltip();
$('[data-toggle="popover"]').popover();
autosize(document.querySelectorAll('textarea'));
})
Test It!
Unfortunately, there isn’t a good way at this time to test the changes made in this step. However, the JS packages will be used in upcoming demos to style the app.
Conclusion
Following the above steps, we have now created a base app for each of the example apps in the demos to build upon.